This problem is also an easy one.
The content is quite short. The difficulty level is 5%.
Project Euler problem #48, “Self Powers”, requires calculating the last 10 digits of the sum of each natural number from 1 to n raised to the power of itself. In other words, we need to compute the following sum:
Since the result of this sum can be extremely large, the key aspect of this problem is to find only the last 10 digits. It is impossible to store the entire result using standard integer variables in most programming languages. Therefore, modular arithmetic should be used to keep track of only the last 10 digits during computation.
The input value is n , and the problem specifically considers the case where n = 1000 . Thus, we need to compute:
and output the last 10 digits of the result.
The most important point in this problem is that only the last 10 digits of the result need to be calculated. Without this constraint, it would be impossible to compute the full value using standard integer types in programming languages. For example, 1000^{1000} has around 3000 digits, and storing it in binary would require approximately 10,000 bits (1,250 bytes).
There are more efficient ways to compute self-powers. For instance, to calculate
However, in this problem, the exponent is at most 1000, so a straightforward, brute-force approach is sufficient.
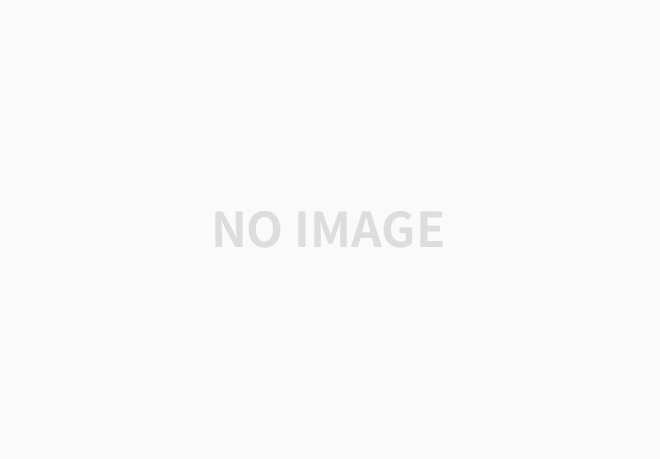
Below is the code I wrote using this simple method.
//------------------------------------------------
// Project Euler #48 - Self Powers
// - by Aubrey Choi
// - created at 2015-03-30
//------------------------------------------------
#include <stdio.h>
#include <stdint.h>
#define DIV 10000000000
int main()
{
uint64_t ans = 0;
for( int i = 1 ; i <= 1000 ; i++ )
{
uint64_t m = 1;
uint64_t s = i;
for( int j = 0 ; j < i ; j++ )
{
m *= s;
m %= DIV;
}
ans += m;
ans %= DIV;
}
printf("ans = %ju\n", ans);
}
'Programming > Project Euler' 카테고리의 다른 글
[C/C++] Project Euler #50 - Consecutive Prime Sum (0) | 2025.03.17 |
---|---|
[C/C++] Project Euler #49 - Prime Permutations (0) | 2025.02.28 |
[C/C++] Project Euler #47 - Distinct Primes Factors (0) | 2025.02.18 |
[C/C++] Project Euler #46 - Goldbach's Other Conjecture (0) | 2025.02.16 |
[C/C++] Project Euler #45 - Triangular, Pentagonal, and Hexagonal (0) | 2025.02.12 |